The HTTP 409 status code is an essential part of the web development ecosystem, particularly in RESTful APIs and client-server communications. It signals that a conflict occurred while processing the request. In this extensive guide, we’ll explore everything about the 409 status code—its meaning, use cases, common causes, fixes, developer implications, server configurations, and answers to popular queries. Whether you’re a beginner or a seasoned developer, this blog covers all angles.
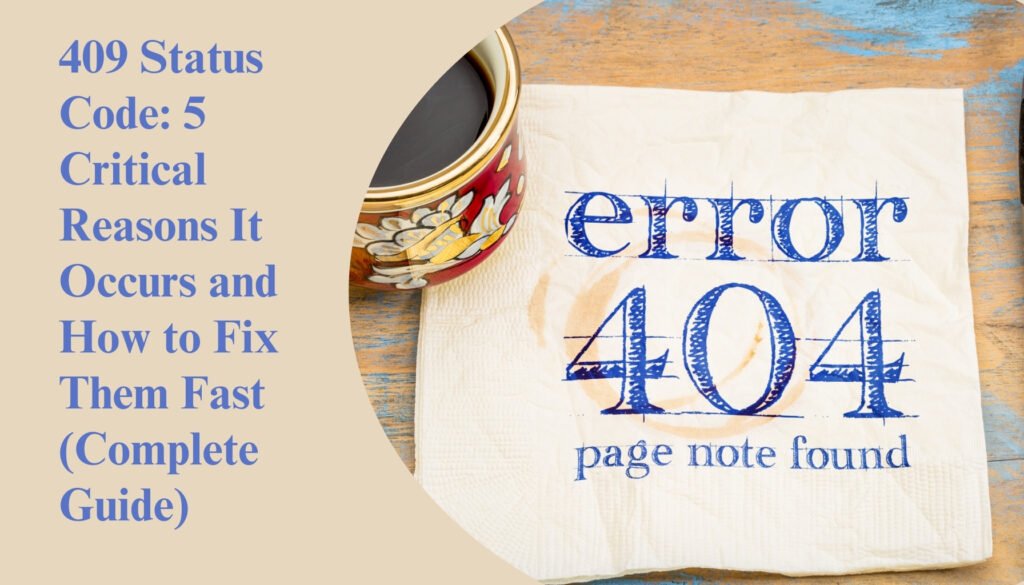
What Is a 409 Status Code?
The 409 status code is an HTTP status code that indicates a conflict. This response is sent when the request cannot be completed because it would result in a conflict with the current state of the target resource. Unlike the 400 Bad Request or 404 Not Found errors, the 409 Conflict implies that the client request is logically correct but cannot be processed due to a conflict.
Technical Definition
According to the RFC 7231 (HTTP/1.1 Semantics and Content):
The 409 (Conflict) status code indicates that the request could not be completed due to a conflict with the current state of the resource.
Key Characteristics of the 409 Status Code
Attribute | Description |
---|---|
Status Code | 409 |
Status Text | Conflict |
Status Type | Client Error |
Usage Context | Conflict between request and resource state |
Common in | REST APIs, Web Applications, Version-controlled environments |
Common Scenarios When 409 Status Code Appears
Here are common use cases and examples where the 409 status code is relevant:
1. Duplicate Resource Creation
Example: A client tries to create a user with a username or email that already exists.
2. Simultaneous Resource Modification (Race Conditions)
Example: Two users edit the same record at the same time.
3. Conflict with Server State
Example: Attempting to update or delete a record that’s already been updated or deleted.
4. PUT or PATCH Requests with Outdated Data
Example: A client sends outdated data that doesn’t match the server version.
5. Business Logic Conflicts
Example: Trying to process a refund on an already refunded order.
Causes of 409 Status Code
Cause Type | Description |
Resource Duplication | Trying to create a record that already exists |
Version Conflicts | Outdated resource version leads to mismatch |
Concurrent Access | Multiple users attempt conflicting changes simultaneously |
Validation Errors | Custom business rules prevent the request |
State Dependency | Client operates on a resource state that no longer exists |
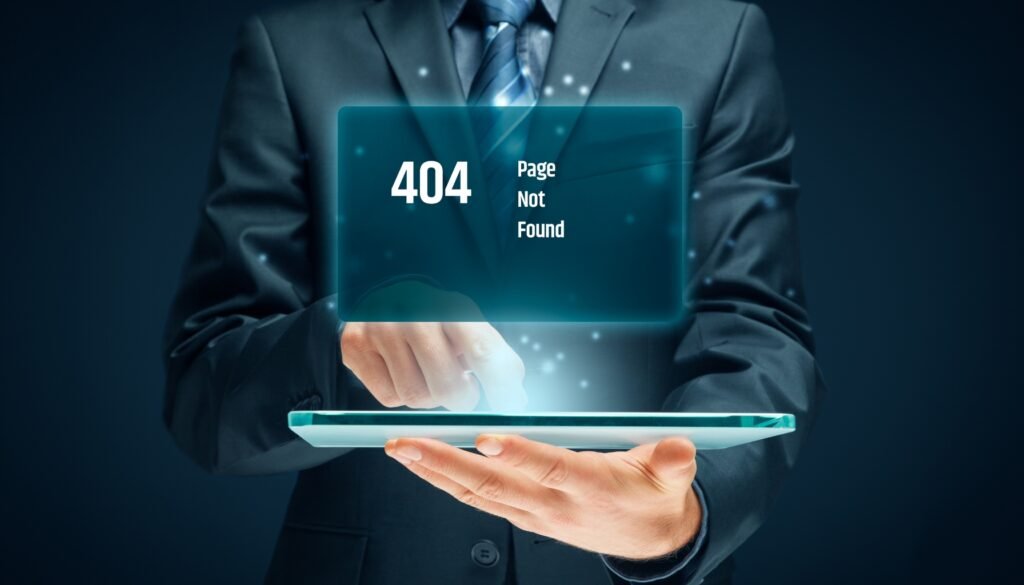
How to Fix 409 Status Code
Fixing a 409 status code requires coordination between the client and server. Here are practical solutions:
A. On the Server Side:
- Use ETags and Versioning: Enforce version control with
ETag
headers andIf-Match
. - Reject Duplicate Resources: Ensure unique constraints and return appropriate errors.
- Custom Merge Logic: Develop a system to merge concurrent updates.
- Maintain Resource Locking: Lock resources temporarily during editing.
B. On the Client Side:
- Implement Retry Mechanisms: Allow clients to retry failed requests.
- Request Confirmation Before Overwriting: Confirm current state before sending changes.
- Avoid Simultaneous Updates: Synchronize with the latest version before submitting.
Server Configuration Examples
Nginx:
location /api/ {
proxy_pass http://backend;
error_page 409 = /custom_409.html;
}
Apache:
ErrorDocument 409 /custom_409.html
Node.js Example:
if (conflictDetected) {
res.status(409).send({ message: 'Conflict detected' });
}
HTTP Status Code Family Comparison
Code | Name | Use Case |
200 | OK | Successful request |
201 | Created | Resource successfully created |
400 | Bad Request | Malformed syntax |
401 | Unauthorized | Authentication required |
403 | Forbidden | No access rights |
404 | Not Found | Resource does not exist |
409 | Conflict | Conflict with current resource state |
412 | Precondition Failed | Failed conditional request |
409 Status Code in RESTful APIs
RESTful APIs use the 409 status code to indicate logical conflicts, such as:
- Duplicate POST requests
- Simultaneous updates using PUT
- Violated unique constraints
- Failed state transitions
Developers must handle 409 errors gracefully and provide users with actionable feedback.
Sample JSON Response for 409 Status Code
{
"status": 409,
"error": "Conflict",
"message": "This username is already taken. Please choose another."
}
People Also Ask (FAQs)
What causes a 409 status code?
A conflict between the client’s request and the server’s current resource state.
Is 409 a server-side or client-side issue?
It’s a client-side error, indicating that the client must modify the request.
Can a 409 status code be retried?
Yes, but only after resolving the conflict (e.g., updating the client-side data).
What is the difference between 409 and 412?
- 409: Conflict due to logical state conflict.
- 412: Failed because a precondition header was not met.
Should I use 409 for duplicates?
Yes, it’s appropriate when duplicates violate resource uniqueness.
People Also Search For
- HTTP status codes list
- REST API error handling
- Difference between 400 and 409
- How to handle conflict in API
- Optimistic locking vs pessimistic locking
- HTTP ETag header
Developer Best Practices
- Use
ETag
and conditional headers likeIf-Match
for versioning. - Inform clients with detailed messages in 409 responses.
- Avoid using 409 for invalid syntax or unauthorized access (use 400 or 401).
- Allow clients to resolve and retry when conflict is detected.
Real-World Applications
Git Version Control
409 errors can occur when attempting to push changes that conflict with the current repository state.
E-Commerce Platforms
Trying to purchase an item that’s already out of stock.
Collaboration Tools
Google Docs or similar tools prevent concurrent overwrites, sometimes returning a 409.
Summary: Why You Must Understand the 409 Status Code
The 409 status code plays a crucial role in preventing data conflicts in modern applications. Whether you manage an e-commerce site, build APIs, or handle version-controlled content, understanding and handling 409 responses ensures smoother user experiences and stronger backend systems.
At Wisdom Digital Marketing, we help businesses and developers optimize their web environments, handle HTTP responses correctly, and build conflict-resistant systems. If your website is running into HTTP errors like the 409 status code, we’re here to help.
Ready to resolve your server issues and streamline your API communication? Visit Wisdom Digital Marketing for expert support and consulting.